Best practices in software development are crucial as they ensure the creation of reliable, maintainable, and scalable software. By adhering to these practices, developers can minimize bugs, reduce technical debt, and enhance the overall quality of the codebase. Best practices promote consistency across teams, facilitate collaboration, and enable easier onboarding of new team members. They also help in anticipating and mitigating potential issues early in the development cycle, leading to more efficient and effective project delivery. Ultimately, best practices are key to building software that meets user expectations and stands the test of time.
Below is a comprehensive guide to some of these practices, along with explanations on why they matter and how to implement them. Feel free to contribute your own ideas and suggestions.
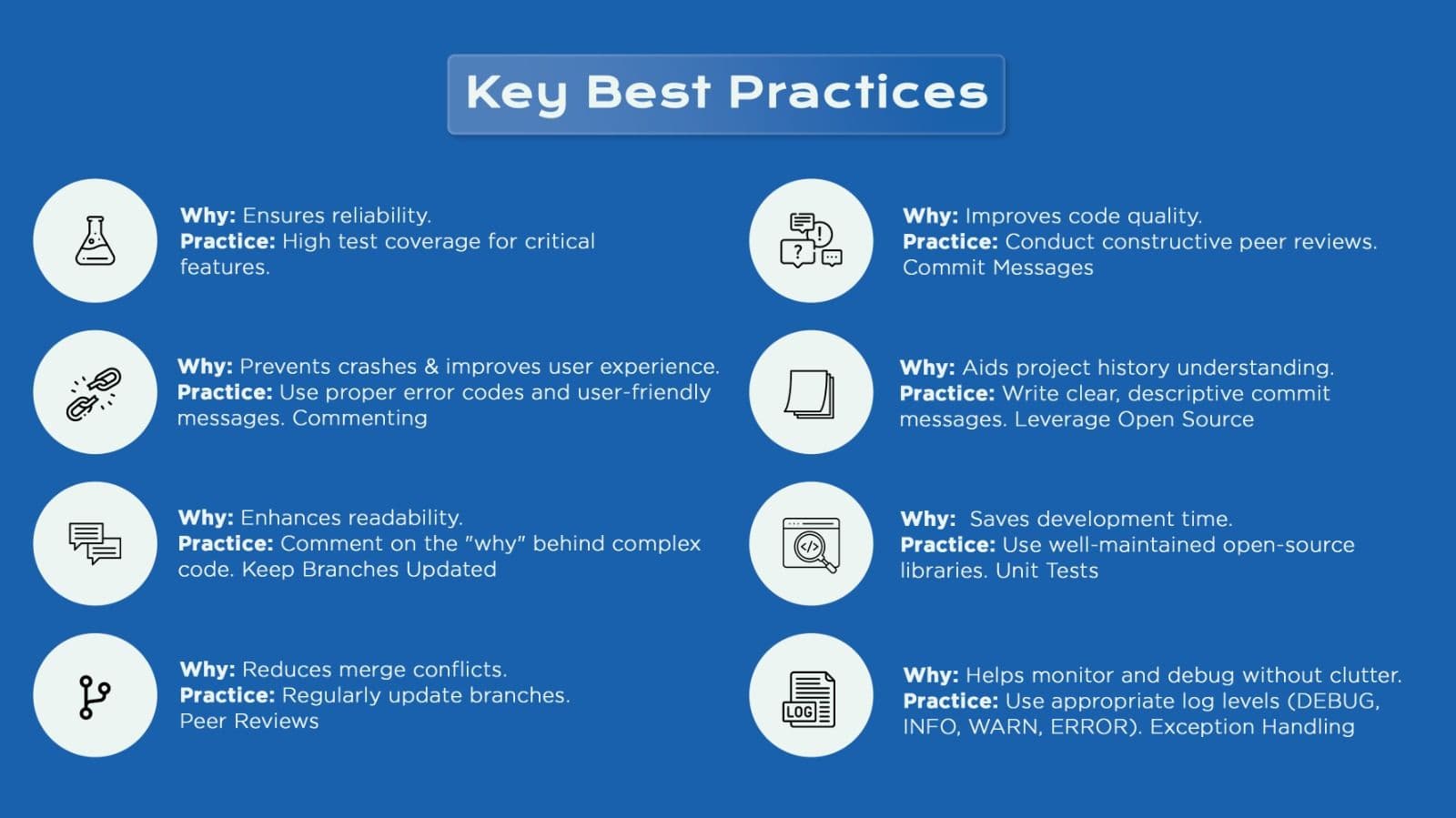
1. Balanced Logging: Finding the Right Level
Why It Matters: Logging is critical for monitoring, debugging, and maintaining our applications. However, too many logs, especially at the INFO level, can clutter log files and make it difficult to find relevant information. Conversely, too few logs can leave us in the dark when troubleshooting issues.
Best Practice: Use DEBUG for detailed information useful during development, INFO for general operational messages, WARN for potentially harmful situations, and ERROR for serious issues that require immediate attention. Avoid logging sensitive information.
Example:
if (user == null) {
logger.warn("User not found for ID: {}", userId);
}
2. Exception Handling: Preventing Crashes and Glitches
Why It Matters: Unhandled exceptions can cause applications to crash, leading to a poor user experience and potential data loss. By managing exceptions effectively, we can prevent these issues and provide meaningful feedback to the user.
Best Practice: Always handle exceptions where they occur and implement a global exception handler to catch any unexpected errors. Ensure that each exception is accompanied by an appropriate error code and user-friendly error message.
Example:
try {
processUserData(user);
} catch (NullPointerException e) {
logger.error("Null value encountered in user data processing", e);
throw new CustomException("User data is incomplete", ERROR_CODE_INVALID_DATA);
}
3. Commenting: Writing Effective Documentation in Code
Why It Matters: Comments are essential for making your code understandable to others (and to yourself in the future). However, excessive or insufficient commenting can be counterproductive. The goal is to make your code self-explanatory, with comments enhancing clarity where necessary.
Best Practice: Write comments that explain the “why” behind complex code, not just the “what.” Keep them concise and to the point, and avoid commenting on obvious code.
Example:
// This loop iterates over the user list to calculate the average age
int totalAge = 0;
for (User user : users) {
totalAge += user.getAge();
}
4. Keep Branches Updated: Avoiding Merge Conflicts
Why It Matters: Outdated branches are a common source of merge conflicts, which can be time-consuming and error-prone to resolve. Regularly updating your branch ensures that you’re working with the latest code and reduces the likelihood of conflicts.
Best Practice: Before starting new work or committing changes, pull the latest updates from the main branch. Make it a habit to rebase or merge frequently to stay in sync.
Example:
git pull origin main
git rebase main
5. Peer Reviews: Learning and Improving Together
Why It Matters: Code reviews are a critical step in maintaining code quality. They allow for shared knowledge, the identification of potential issues, and the opportunity to learn from each other. This practice also helps to catch errors that might have been overlooked.
Best Practice: Conduct peer reviews for every significant code change. Be constructive in your feedback, focusing on the code rather than the coder. Use reviews as a learning opportunity for both the reviewer and the author.
Example:
Reviewer Comment: “Consider using a StringBuilder here to improve performance when concatenating strings in a loop.”
6. Commit Messages: Communicating Your Changes
Why It Matters: A good commit message explains what has been changed and why. This is crucial for understanding the history of a project and for debugging purposes. Clear commit messages make it easier for others (and your future self) to understand the context of changes.
Best Practice: Write concise, descriptive commit messages that explain the rationale behind changes. Use a consistent format, typically a short summary followed by a more detailed explanation if necessary.
Example:
git commit -m "Fix NullPointerException in user data processing"
git commit -m "Add error handling for missing user fields during data processing"
7. Leverage Open Source: Don’t Reinvent the Wheel
Why It Matters: Open-source libraries can save significant development time and effort by providing pre-built functionality that you can integrate into your projects. However, it’s important to use them wisely, ensuring they are well-maintained and licensed appropriately.
Best Practice: Before implementing new functionality, check if a reliable open-source library exists that meets your needs. Ensure that any third-party libraries you use are compatible with your project’s license (e.g., Apache 2.0).
Example:
Use Case: Instead of writing a custom JSON parser, consider using Jackson or Gson.
8. Write Unit Tests: Ensuring Code Reliability
Why It Matters: Unit tests validate that individual components of your application work as expected. They help prevent bugs from being introduced and provide a safety net when refactoring code. Without tests, it’s harder to ensure that changes haven’t broken existing functionality.
Best Practice: Write unit tests for all new features and bug fixes. Aim for high test coverage, focusing on critical and complex parts of the application. Use mocking frameworks to isolate the code under test.
Example:
@Test
public void testCalculateAverageAge() {
List<User> users = Arrays.asList(new User(25), new User(30), new User(35));
int averageAge = userService.calculateAverageAge(users);
assertEquals(30, averageAge);
}
9. API Management: Organizing Your API Testing
Why It Matters: APIs are often the backbone of your application, and maintaining a clear, organized collection of them is essential for efficient testing and collaboration. Postman collections help you document, test, and share your APIs easily.
Best Practice: Maintain an up-to-date Postman collection of your APIs in your local workspace. This makes it easier to test APIs during development and ensures that your documentation is always current.
Example:
Action: Create and organize Postman folders by feature or module, including example requests and responses.
10. Git Proficiency: Mastering the Essential Commands
Why It Matters: Git is a powerful tool, and knowing how to use it effectively can save time and prevent issues during development. Commands like git log, cherry-pick, and stash allow you to manage your changes more efficiently and recover from mistakes.
Best Practice: Take the time to learn and practice key Git commands. They will become invaluable in your day-to-day development tasks, especially when dealing with complex version control scenarios.
Example:
git log: View the history of commits
git cherry-pick: Apply a specific commit from one branch to another
git stash: Temporarily save changes that are not ready to be committed
11. Performance Considerations: Coding with an Eye on Efficiency
Why It Matters: Performance issues can significantly impact the user experience and scalability of your application. By considering performance during development, you can prevent bottlenecks and ensure your application runs smoothly under load.
Best Practice: Pay attention to performance as you code. Optimize database queries with indexes, avoid unnecessary synchronization in multi-threaded environments, and monitor for potential memory leaks.
Example:
// Example of using an index to optimize a database query
@Query("SELECT u FROM User u WHERE u.email = :email")
List<User> findByEmail(@Param("email") String email);
12. Optimize JVM: Managing Resources Efficiently
Why It Matters: The Java Virtual Machine (JVM) is the runtime environment for Java applications, and managing its resources effectively is key to ensuring optimal performance. Connection and thread pools, for example, can greatly enhance efficiency and reduce latency.
Best Practice: Implement connection pools and thread pools to manage resources efficiently. This reduces the overhead of repeatedly creating and destroying objects, leading to better performance.
Example:
// Example of setting up a connection pool using HikariCP
HikariConfig config = new HikariConfig();
config.setJdbcUrl("jdbc:mysql://localhost:3306/mydb");
config.setUsername("user");
config.setPassword("password");
HikariDataSource dataSource = new HikariDataSource(config);
13. Caching Strategies: Improving Performance While Avoiding Stale Data
Why It Matters: Caching can significantly improve the performance of your application by reducing the need to repeatedly fetch the same data. However, without proper invalidation strategies, you risk serving outdated or incorrect data to users.
Best Practice: Implement caching for frequently accessed data, but ensure that you have a reliable invalidation mechanism in place. This prevents stale data from being served and keeps the user experience consistent.
Example:
@Cacheable(value = "userCache", key = "#userId")
public User getUserById(Long userId) {
return userRepository.findById(userId).orElse(null);
}
@CacheEvict(value = "userCache", key = "#userId")
public void updateUser(User user) {
userRepository.save(user);
}
By following these best practices, we can ensure that our code remains high-quality, maintainable, and efficient. Let’s continue to learn from each other and improve our development processes.